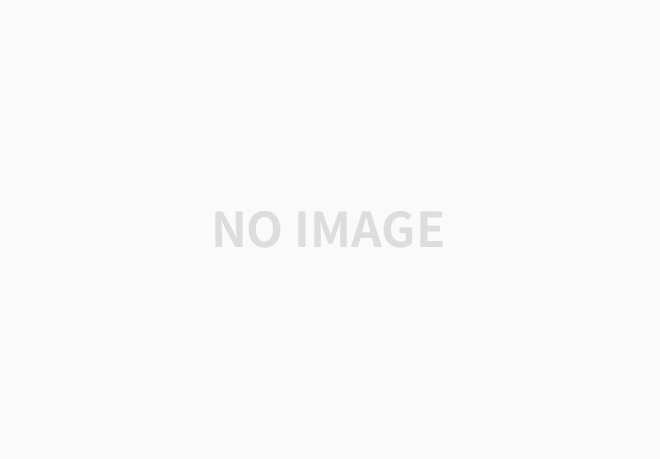
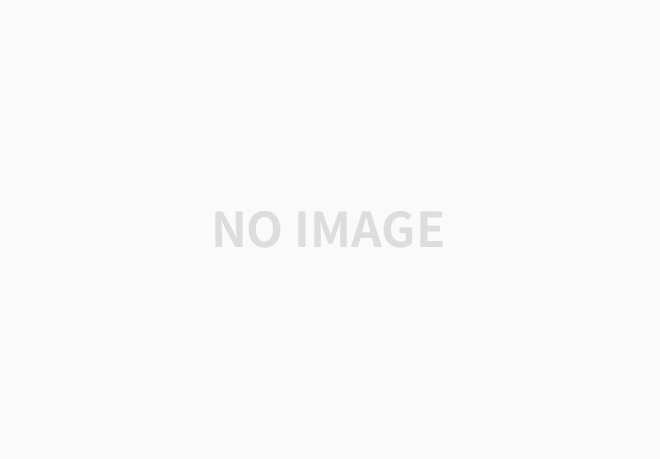
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main(void) {
int x;
scanf("%d", &x);
int n = 1;
int position = 0;
while (position + n < x) {
position += n;
n += 1;
}
int r_position = x - position;
int 분자, 분모;
if (n % 2 == 0) {
분자 = r_position;
분모 = n - r_position + 1;
}
else {
분자 = n - r_position + 1;;
분모 = r_position;
}
printf("%d/%d\n", 분자, 분모);
return 0;
}
1,문제에서 말하는 x의 값을 입력받는다.
2.while문을 통하여 현재 대각선의 위치가 어디인지 확인한다.
2-1.이때 대각선의 수를 찾는 방법은 1+2+3+4.... 를 하나하나 더해서 찾는 방식이다.
3,분자와 분모를 확인한다,
3-1.이때 홀수 대각선인지 짝수 대각선인지에 따라 분모와 분자가 증가하는 방식이 달라진다.
4. 위 조건을 고려하여 분자와 분모를 확인 후 출력한다.
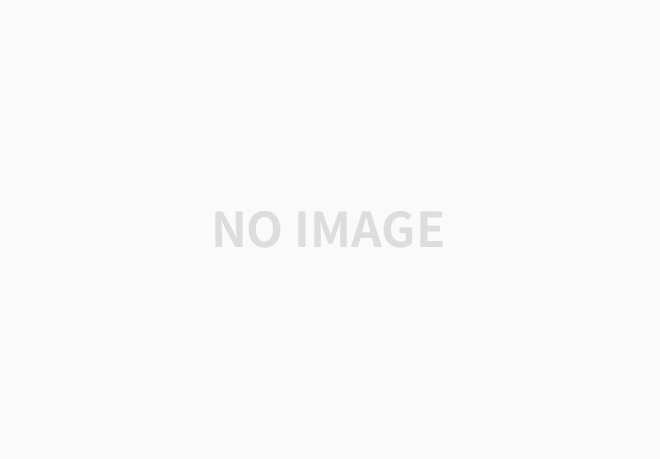
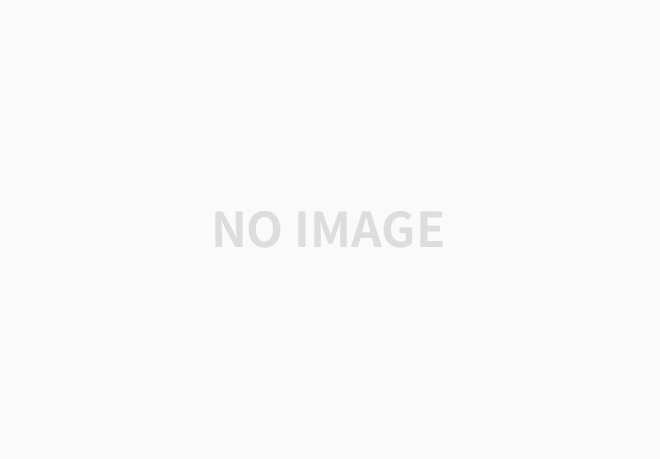
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int compare(const void* a, const void* b) {
char* wordA = *(char**)a;
char* wordB = *(char**)b;
int lenA = strlen(wordA);
int lenB = strlen(wordB);
if (lenA != lenB) {
return lenA - lenB;
}
return strcmp(wordA, wordB);
}
int main(void) {
int n;
char* word[20000];
char buffer[51];
scanf("%d", &n);
for (int i = 0; i < n; i++) {
word[i] = (char*)malloc(51 * sizeof(char));
scanf("%s", word[i]);
}
qsort(word, n, sizeof(char*), compare);
printf("%s\n", word[0]);
for (int i = 1; i < n; i++) {
if (strcmp(word[i], word[i - 1]) != 0) {
printf("%s\n", word[i]);
}
}
for (int i = 0; i < n; i++) {
free(word[i]);
}
return 0;
}
1.단어의 수를 입력받고 단어들의 배열, 각 칸에 들어갈 배열을 또 따로 만든다. (2차원 배열 느낌)
2.단어를 입력받을 땐 malloc을 사용하여 입력을 받는다.
3.qosrt함수를 사용한다. 이때 단어의 길이가 같을 때를 고려하여 정렬한다.
4. 정렬된 단어 배열을 출력한다. 이때 이미 나왔던 단어인지 고려한다.
5.malloc으로 할당했던 메모리 공간을 free로 해지한다.